@jero
Another option, just while it is in my head so I donât forget, is to create one flow that just has the webhook and saves the entries to a table.
Then create another flow that uses a schedule as the trigger to pull the data from the table and check if the date is older than 30 days. If so, send an email. If false, because the flow is on a schedule, it will keep checking until it is true.
Anyway, as I said, I will think about it later.
This is my updated reply after a lot of thinking; I apologise in advance for the lengthy reply.
@jero
The way I see it is that I only see two possible options from here:
1. Add additional delay steps, as I noted it states on the step itself:
Note: The maximum duration per step is 30 days.
So theoretically, this would allow you to overcome the 30-day max period, although I am unsure if this is even allowed, frowned upon, will actually work, or is even a viable solution. Not to mention that it wouldnât really be of much use to you anyway, as ultimately, you cannot restart/re-trigger the flow to be able to then run the conditional logic again!
I did think about suggesting that you add more branches (nest) inside of the FALSE branch, which would allow you to make additional decisions based on newer logic. I am not sure if you are aware that you can do this; see the links below for further info:
But again, I canât see how these will help, as you want to restart the flow!



The other thing bothering me, and I am trying to understand it, is that you mentioned that each time an event is booked, it triggers the webhook with the event date.
So can I ask, if this is the case, then how would you be able to go back and check a prior booking after a delay? Surely, as soon as a second booking happens and the webhook is triggered, the first date will be wiped out by the new value, as in your current flow, I cannot see it being stored anywhere.
2. The most viable solution I can think of right now is to create two separate flows. (Think of them like one flow, but split into two parts).
The first flow can go one of two ways:
-
a) The first approach will simply have your webhook trigger and a table or storage piece (or, of course, if you prefer, a Google sheet, notion, airtable, or whatever) to store the data from the webhook into a table.
-
b) The second approach is a little more like what you have now, but instead of using the delay piece, if the date equates to FALSE, swap this out for a table piece to store the data of only the dates that are not older than 30 days.
Note: As I donât know what data you are working with, it is hard to give a full solution. You may very well need multiple columns.
For Example:
customer_email, booking_id, event_date
Example Table:
Note: You could even take this a step further and have event_start_date
, event_end_date
, etc. This would allow for easier conditional checking down the line to determine if the date is older than 30 days. (But for now, I will leave that up to you so I can keep this example simplified.)
Extra Note: Taking approach b) would allow you to instantly send an email to any booking where the end date condition has been met (is TRUE) and then store the dates where the condition hasnât been met (is FALSE) for a later check in the separate flow, which we will touch on below.
So, in the second flow, you could start with a Scheduled trigger, which will allow you to set the flow to run every X number of days.
Similar to the first flow above (but now without the need for the webhook trigger), you can now simply fetch your data from the table to automatically compare the dates for the date checking to determine whether or not to send an email (for this step, you could actually utilise the loop piece to iterate through each entry in the table).
Then, as before, re-use your router piece to send the email if the condition is TRUE.
Important: Here, I would also add some step after sending the email to remove that entry from the table, just to make sure that it wonât be sent again the next time the scheduled flow runs.
Note: If you wanted to complicate things, you could, in theory, add a new column that has 'success'
, 'sent'
, or some other flag, then add extra conditional checks to the router step so that the next time the scheduled flow runs, it knows to ignore those entries in the table as they have already had their email sent out. But it seems a bit overly complex and unnecessary, so it would just be easier to remove the entry entirely after the email has been sent).
If it equates to FALSE, then do nothing (so just leave it blank with no steps; then, when it re-runs again the next time, at some point, the condition will eventually become true, and the email will be sent out).
Optional Approach:
As we mentioned earlier, it is worth noting you could replace the scheduled approach with the sub-flow approach. This would allow you to call on one of the flows from the other, but as far as I know, as stated in the documentation on it:
With the SubFlows piece, weâve made calling additional flows as simple as selecting an option from a dropdown menu. Whether you want to wait for the subflowâs response and act based on that or simply call the subflow without waiting for a response, the SubFlows piece gives you the flexibility to do either.
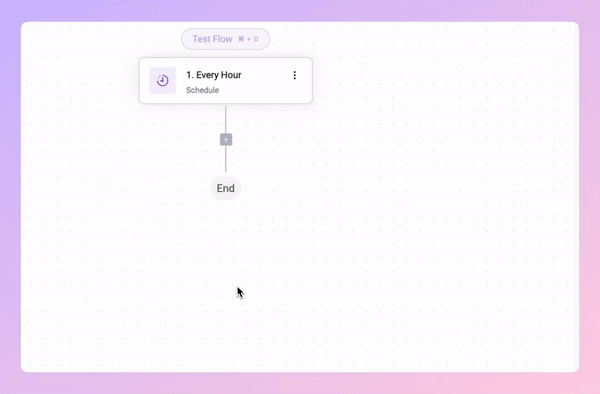
To ensure ease of use, the only subflows that will appear in the dropdown menu are those that meet two conditions:
- The subflow must have a âCallable Flowâ trigger.
- The subflow must be published.
This ensures users are only selecting valid subflows, keeping the process intuitive and hassle-free.
Documentation Links:
So you would need to utilise one of the triggers for the Sub Flow piece.
So my thinking here was to take the same approach as the above, but rather than using the schedule, you can now add a step to call on the second sub flow to pull the data from the tables and check it.
The only downside to this approach would be that it would only get triggered each time a new booking happens when the webhook is called, so it might not be the best option if you donât get a booking for 90+ days, that means any of the entries in the table, wonât get checked for those 90+ days either.
Which is why, I decided on it being a secondary solution.
Hopefully, this will help you in some way to achieve what you are trying to achieve. Or even spark that âA-haâ moment, and you will come up with something that works for you.
I guess this could be a good next step for ActivePieces (@Abdul): incorporating a way in AP for users to retrigger an entire flow from inside a step, like for your needed use case above. (Maybe it is already possible, and I just donât know about it.)
Kind regards,